The Windows 7 taskbar does this nifty little background animation when you mouse over an open application’s icon. I wanted to see if the effect could be duplicated on a web page.
Suppose we have a couple of inline elements:
<div> <img class="bgmove" src="wsbadge-empty.png"> </div>
<h1> <span class="bgmove">Packets!</span> </h1>
The image is mostly transparent:
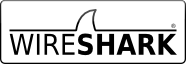
We have a background that’s slightly larger than our elements, which lets us position it using negative offsets:
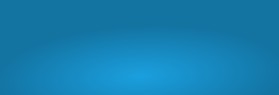
.bgmove { background-image: url('wsbadge-bg.png'); background-repeat: no-repeat; background-position: -50px -20px; }
jQuery lets us do two important things: track mouse motion and determine an element’s dimensions. This lets us shift the backround around when we mouse over each element:
$(document).ready(function(){ $("div img.bgmove").pngFix(); $(".bgmove").mousemove(function(e){ var elWidth = $(e.target).outerWidth(); var elHeight = $(e.target).outerHeight(); var bgWidth = 279; var bgHeight = 95; var x = e.pageX - this.offsetLeft; var y = e.pageY - this.offsetTop; //var offX = -1 * (x * (bgWidth - elWidth) / elWidth); // Against the mouse var offX = (x * (bgWidth - elWidth) / elWidth) - (bgWidth - elWidth); // With the mouse var offY = -1 * (y * (bgHeight - elHeight) / elHeight); // Against the mouse //var offY = (y * (bgHeight - elHeight) / elHeight) - (bgHeight - elHeight); // With the mouse bgPos = offX + 'px ' + offY + 'px'; this.style.backgroundPosition = bgPos; }); })
You can see it all in action on the demo page. Combining this with jQuery animation is left as an exercise for the reader. The effect works in Firefox, Safari, Chrome, and IE 7+. IE 6 has trouble with the transparent PNG.